Hi,
I’m working on blender plugin which export probes (irradiance grid and reflection cubemap). It is still in progress but it has enough features to be integrated with existing engine.
I didn’t see much stuff about threejs supporting light probes it so I would like to work also on some plugin which extends existing light system with probes. Is anyone is also interested to work on something like this or know existing work ?
Cheers
2 Likes
Mugen87
September 13, 2023, 2:51pm
2
three.js
has a basic light probe implementation. There is the LightProbe class itself as well as a LightProbeGenerator that can be used to generate a light probe from cube textures/render targets.
There is also a WIP PR by @donmccurdy which adds a LightProbeVolume
class.
mrdoob:dev
← donmccurdy:feat-lightprobevolume
opened 09:26PM - 11 Jan 20 UTC
## Summary
A LightProbeVolume samples diffuse indirect lighting in a scene at… each of several LightProbe locations, then provides approximate diffuse lighting for dynamic objects at any location within that space. The method complements baked lighting and lightmaps — which only support static objects — by providing high-quality lighting for dynamic objects as they move throughout a larger scene. Like lightmaps, LightProbeVolumes can be 'baked' offline and stored, then loaded and applied at runtime very efficiently.
Fixes https://github.com/mrdoob/three.js/issues/16228.
Thanks to @WestLangley, @bhouston, and @richardmonette for helpful discussions and code that contributed to this PR.
## API
Bake:
```javascript
var cubeCamera = new THREE.CubeCamera( 0.1, 100, 256, {
format: THREE.RGBAFormat,
magFilter: THREE.LinearFilter,
minFilter: THREE.LinearFilter
} );
// Renderer output is sRGB. Configure renderTarget accordingly.
cubeCamera.renderTarget.texture.encoding = THREE.sRGBEncoding;
// Generate a light probe volume and populate probes in a 10x3x10 grid. Then
// sample lighting at each probe using the CubeCamera.
var volume = new THREE.LightProbeVolume()
.setFromBounds( new THREE.Box3().setFromObject( scene ), 10, 3, 10 )
.build()
.bake( renderer, scene, cubeCamera );
// Store volume and baked lighting as a small JSON object.
var data = volume.toJSON();
```
Apply:
```javascript
// Reconstruct the volume with pre-baked lighting.
var volume = new THREE.LightProbeVolume().fromJSON( data );
scene.add( volume );
probe = new THREE.LightProbe();
scene.add( probe );
function render () {
volume.update( mesh, probe.sh );
renderer.render( scene, camera );
}
```
Lighting is sampled only at the center of each mesh, and larger objects like terrain and buildings will not receive realistic lighting from a single sample. Shadows are not cast by light probes.
## Unresolved
- [ ] **Per-object SH.** While per-object SH coordinates can be computed, only one "probe" is actually in the scene graph functioning as a light. So only one mesh can receive dynamic lighting. This can be resolved with an API to assign SH coordinates or a light probe to a specific object or material.
- [ ] **Optimize.** Various inefficiencies left as TODO. For example, LightProbeVolumeHelper should use InstancedMesh, and `volume.update()` should search incrementally from the last known cell to improve update times.
- [ ] **Correct baking.** I'm not convinced my "baking" method is quite right, but it looks pretty good. Maybe this could be done offline in other tools.
- [X] **Class structure.** ~~In this demo, LightProbeVolume is in the scene graph but its probes are not (I don't want them all affecting the mesh). I'm open to other ways of structuring this.~~ It's useful for the volume to have position in the scene.
- [x] **Grid volumes.** ~~Should we allow arbitrarily-shaped volumes, or require grid-shaped volumes? Blender and Godot appear to be OK with grids. That would let us omit the Delaunay library (which generates ~5x~ 1.5x more tetrahedra than needed, for a grid?), improving volume creation time. And if (in the future) we want to upload the whole volume's SH coordinates to a GPU texture for sampling in the fragment shader, I think a grid would be necessary. But it is less flexible / optimizable.~~ Grids only, for now.
## Demo
https://raw.githack.com/donmccurdy/three.js/feat-lightprobevolume-build/examples/?q=volume#webgl_lightprobe_volume
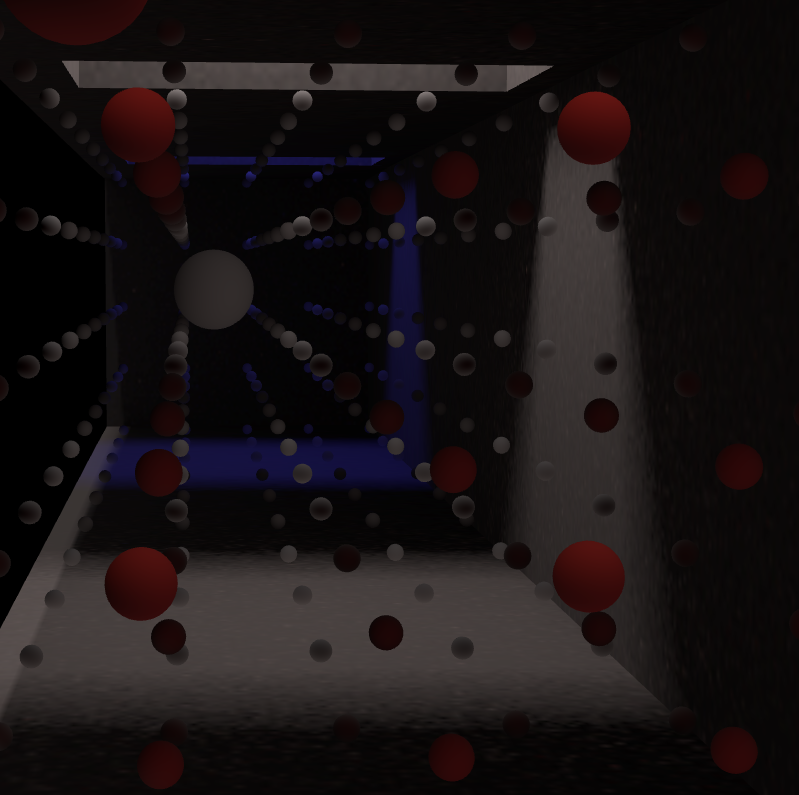
I think it’s best if you use this implementation as a basis for your exporter since LightProbeVolume
can read irradiance data from JSON files. It would be great if your Blender exporter could support the respective JSON format.
3 Likes
Thanks, really interesting stuff.
I forgot to share the link of the blender plugin repo. It works out the box by just installing the plugin source in blender.
for info It exports :
irradiance probes grid as cubemaps sheet (one sheet per grid, SH not supported yet)
and reflection cubemap as multi level roughness level cubemap sheet.
6 Likes