I have been using three.js for couple of years for our project. The version that i am using is r 97. It looks like Three js is migrated to es6. Now i want to use the latest but i dont know how do i do this. Looks like babel is one such solution where one can transform es6 to prototyping form. We use require, define properties to create class and i was also extending some of the Three Objects (THREE.Object for example ) according to the requirement. Its not possible for me completely rewrite my code into es6. Any help would be great?? Any example on how to convert THREE es6 code into earlier propotyping format would be very helpful.
The same question was lately asked at GitHub. The issue provides a solution for producing ES5 builds.
opened 03:27PM - 19 Jun 21 UTC
closed 03:57PM - 19 Jun 21 UTC
Help (please use the forum)
**Is your feature request related to a problem? Please describe.**
The current … UMD package is ES6. If my code is Es5, I can't inherit three related classes
```js
var __extends = (this && this.__extends) || (function () {
var extendStatics = function (d, b) {
extendStatics = Object.setPrototypeOf ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||
function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };
return extendStatics(d, b);
};
return function (d, b) {
extendStatics(d, b);
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
};
})();
/**
* @author WestLangley / http://github.com/WestLangley
*
*/
import * as THREE from 'three';
import LineSegmentsGeometry from './LineSegmentsGeometry';
import { addAttribute } from '../ThreeAdaptUtil';
import LineMaterial from './LineMaterial';
var LineSegments2 = /** @class */ (function (_super) {
__extends(LineSegments2, _super);
function LineSegments2(geometry, material) {
var _this = _super.call(this, geometry, material) || this;
_this.isLineSegments2 = true;
_this.type = 'LineSegments2';
_this.geometry = geometry !== undefined ? geometry : new LineSegmentsGeometry();
_this.material = material !== undefined ? material : new LineMaterial({ color: Math.random() * 0xffffff });
return _this;
}
LineSegments2.prototype.computeLineDistances = function () {
var start = new THREE.Vector3();
var end = new THREE.Vector3();
var geometry = this.geometry;
var instanceStart = geometry.attributes.instanceStart;
var instanceEnd = geometry.attributes.instanceEnd;
var lineDistances = new Float32Array(2 * instanceStart.data.count);
for (var i = 0, j = 0, l = instanceStart.data.count; i < l; i++, j += 2) {
start.fromBufferAttribute(instanceStart, i);
end.fromBufferAttribute(instanceEnd, i);
lineDistances[j] = (j === 0) ? 0 : lineDistances[j - 1];
lineDistances[j + 1] = lineDistances[j] + start.distanceTo(end);
}
var instanceDistanceBuffer = new THREE.InstancedInterleavedBuffer(lineDistances, 2, 1); // d0, d1
addAttribute(geometry, 'instanceDistanceStart', new THREE.InterleavedBufferAttribute(instanceDistanceBuffer, 1, 0));
addAttribute(geometry, 'instanceDistanceEnd', new THREE.InterleavedBufferAttribute(instanceDistanceBuffer, 1, 1));
// geometry.addAttribute('instanceDistanceStart', new THREE.InterleavedBufferAttribute(instanceDistanceBuffer, 1, 0)); // d0
// geometry.addAttribute('instanceDistanceEnd', new THREE.InterleavedBufferAttribute(instanceDistanceBuffer, 1, 1)); // d1
return this;
};
// eslint-disable-next-line no-unused-vars
LineSegments2.prototype.copy = function (source) {
// todo
return this;
};
return LineSegments2;
}(THREE.Mesh));
;
// LineSegments2.prototype = Object.assign(Object.create(THREE.Mesh.prototype), {
// constructor: LineSegments2,
// isLineSegments2: true,
// });
export default LineSegments2;
//# sourceMappingURL=LineSegments2.js.map
```
https://cdn.jsdelivr.net/npm/three@0.129.0/build/three.js
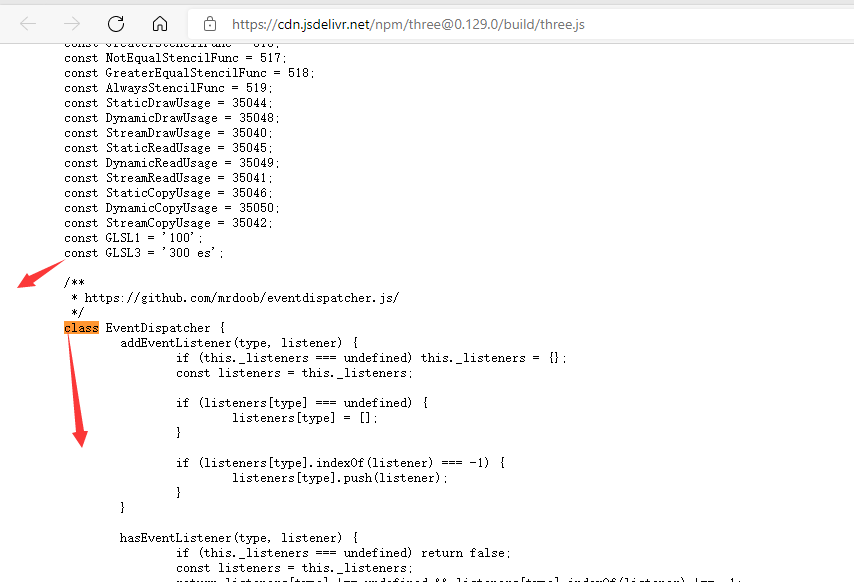
A clear and concise description of what the problem is. Ex. I'm always frustrated when [...]
**Describe the solution you'd like**
umd package Use Es5 version, The ESM package can use ES6
A clear and concise description of what you want to happen.
**Describe alternatives you've considered**
A clear and concise description of any alternative solutions or features you've considered.
**Additional context**
Add any other context or screenshots about the feature request here.